When To Use Generics in Golang
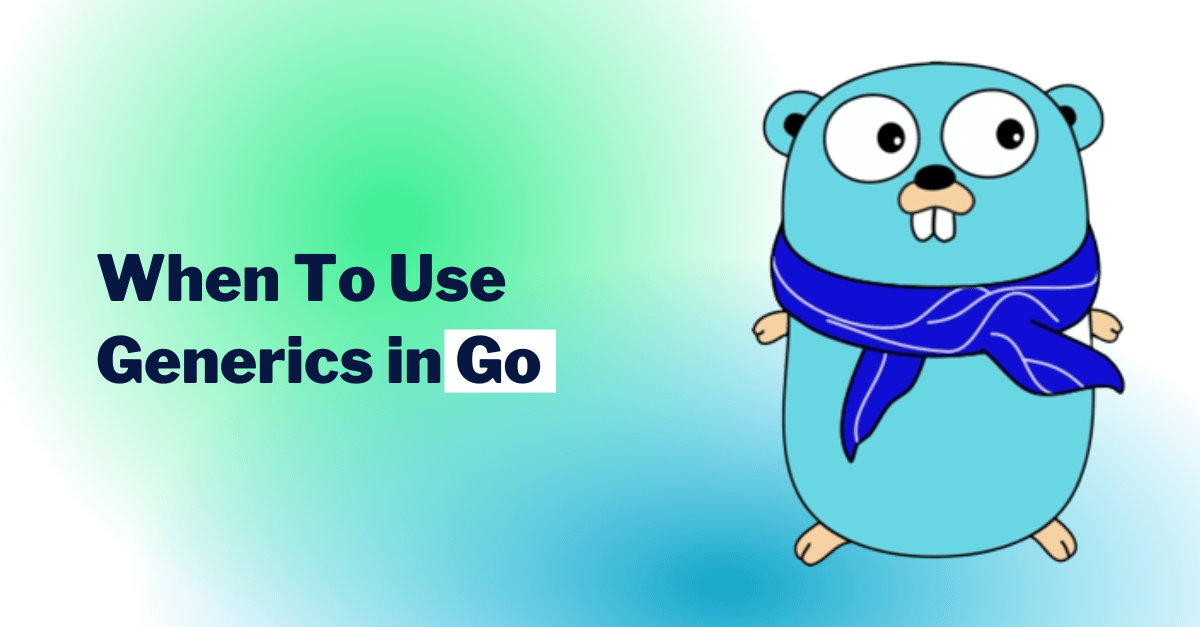
Before the Go Programming Language 1.18 release, generic was a very serious problem for Golang Developers. I understand that you may find it strange because you may not be more familiar with what Generics are. I'll be sharing knowledge about generics in this blog, including When to Use It and How to Use It. So let's start with learning Generics.
Prerequisites
To properly understand the principles outlined in this blog, I would like to ask that you read over the concepts I will be mentioning below before continuing.
- Pointers: In Go, pointers are frequently used in generics. They let you provide variables by reference rather than by value. It's crucial to comprehend how pointers function and how to use them properly.
- Interfaces: Generics in Go are often implemented using the interface{} type and type assertions. To understand how this works, you should have a good understanding of Go's interface type and how to use it.
- Type assertions: Type assertions allow you to convert an interface{} value to a specific type. To understand how generics work in Go, you should be familiar with the syntax and behavior of type assertions.
- Reflection: Reflection, which enables you to view a variable's type and value at runtime, is frequently used with generics in Go. You need to have a fundamental understanding of how reflection functions and how to use it in order to comprehend how generics in Go operate.
- Having a solid grasp of Go's type system, including ideas like type inference, type conversion, and type aliasing, is also beneficial.
- You should be well-prepared to start learning about generics in Go if you are familiar with these ideas and are at ease developing Go code.
Generics in Go
In Go, generics refer to the ability to write functions or types that can work with multiple types rather than being specific to one type. This allows you to write more general, reusable code that can be used with a variety of different types. Generics in Go are implemented using the interface{} type and type assertions. This means that you can write a function or type that takes or returns the interface{} type, and then use type assertions to convert values of this type to a specific type when needed. Please refer to Image 1 for the example for better clarification.

Any two variables can have their values switched using this function as long as they are of the same type. You would call this function as follows to swap the values of the two variables x and y. Please refer to Image 2,

When to Implement Generic?
Generics should generally be used in Go when writing code that can operate on several types as opposed to being restricted to only one. This can make it simpler for you to create more flexible, broad, and reusable code.
Here are some specific instances in Go where you might think about using generics:
- When you need to create a type or function that can consistently operate on a variety of types. For instance, you could create a generic function that accepts two arguments and always returns the greater of the two.
- When you don't want to write different versions of a function or type for each type and instead want to develop one that can work with multiple types, as an illustration, you could create a generic sorting function that can organize slices of any kind.
- When you want to write a function or type that can work with multiple types, but you don't know ahead of time which types it will be working with. For example, you might write a generic function that takes an interface{} argument and returns a boolean indicating whether the argument is a zero value for its type.
Generic might not be the best option in some circumstances. For instance, you might not need to utilize generics if you are working with a limited, fixed collection of types and you do not need to build general, reusable code. In general, before utilizing generics in your code base, it's a good idea to assess whether they will help you produce cleaner, more maintainable code. Start with some simpler examples if you're unfamiliar with Go and generics, then progress to more sophisticated usage of generics. Now, let us understand the limitation of using Generics in a detailed way to making the understanding more clear and accurate.
Limitations for using Generics
Here, The limits of Generics in Go will be discussed. When preparing for Golang programming, there are some limits that, in my opinion, shouldn't be ignored. I've listed them below.
- When creating a function or type in Go, a type parameter cannot be specified. This implies that you cannot develop a generic function or type that functions with any type, unlike in several other languages. Instead, you must make use of the type and type assertions of the interface to create generic functions and types that can communicate with a variety of types.
- Go does not provide a reflection on types or values that have a type of interface. This implies that you are unable to use reflection to check the type or value of a variable with an interface type or to activate methods on such a variable.
- Type-based overloading of functions and methods is not supported in Go. As a result, you cannot declare multiple instances of a function or method bearing the same name but with different parameters or return values.
Conclusion
Generics can be a powerful tool for writing reusable, flexible code in Go. They allow you to write functions or types that can work with multiple types rather than being specific to one type, which can help you write more general, maintainable code. Some situations where you might consider using generics in Go include when you want to write a function or type that can operate on multiple types in a consistent way, when you want to write a function or type that can work with multiple types but don't want to write separate versions for each type, or when you want to write a function or type that can work with multiple types but don't know ahead of time which types it will be working with. However, generics may not be the best choice in all situations, and it's a good idea to consider whether they will help you write cleaner, more maintainable code before using them in your codebase. Hope this explanation blog was helpful for your learning.

Rishav’s professional life began in 2019 when he completed his B. Tech in computer science and engineering. He has been in content development for the last 2.5 years. Tech is not his profession, it’s his curiosity that he believes to explore as much as he can.